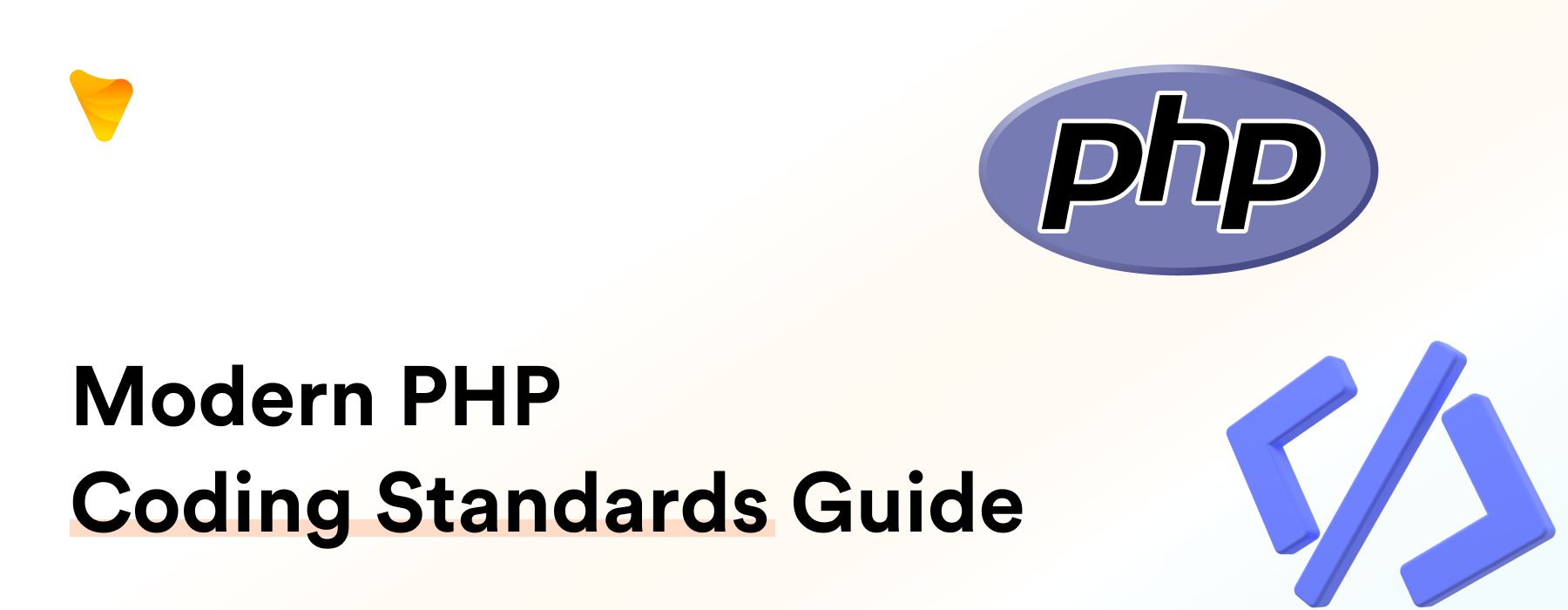
PHP Coding Standards and Style Guides: A Complete Guide to Code in a Modern Way
Introduction
The Need for PHP Coding Standards & Style Guides
Consistent coding habits, including proper indenting and adherence to PSR-1 standards in PHP documents, are essential for order in digital development, especially in team setups. PSR-12 style guides enhance readability and reduce logic errors, emphasizing deliberate variable interpolation. Referencing multiple standards ensures cohesive use of PHP tags.
Dive into a Modern Way of Coding
Adopting a modern way of coding not only improves readability but also bolsters efficiency. This guide will help you unravel the secrets to tasteful and effective PHP coding. So, brace up and let’s sail the seas of modern PHP coding.
Basic PHP Coding Standards
Naming Conventions for Variables, Functions and Classes
In coding, meaningful names like $app_config and adherence to conventions (e.g., snake_case) enhance clarity. Prioritize lowerCamelCase, capitalize acronyms (CLASSNAME), and employ clear type casting (e.g., (int) over (integer)). Use parentheses judiciously, avoid single-letter variables, and format division comments with asterisks. Embrace grouping in import use statements, prefix functions with module names, and prioritize clarity over brevity for effective PHP coding.
File Organization & Structure
A well-organized file structure goes a long way in maintaining clarity in your PHP projects. Name your files meaningfully, with lowercase letters, separating words with a hyphen. For instance, use autoloader.php or app-config.php. It’s important to store them accurately in the relevant directory.
Follow these mentioned formatting guidelines:
- Set character encoding to UTF-8 without BOM through Sublime.app → File › Save with Encoding › UTF-8.
- Ensure the file header has the correct settings.
- Use Unix (LF) line endings by clicking Sublime.app → View › Line Endings › Unix for proper alignment.
Improving your understanding of file organization, which involves appropriate utilization of headers and directories, aids in maintaining the readability and managing your PHP projects precisely. Always remember – in the realm of coding – a tidy project is definitely a joyful project!
Moreover, these tips could be of great help when working with frameworks like Zend. The usage of correct headers and proper alignment can prevent unwanted errors like “header already sent” and XHTML/XML validation issues.
PHP Commenting Practices
Single-line and Multi-lines Comments
PHP allows you to weave comments into your code. This proves helpful for maintaining coherence and enhancing understanding among your team but avoids the use of shorthand due to PHP start tags. Single-line comments require two forward slashes, and importantly, there’s no need for shorthand here. For example, // This is a single line comment.
When it comes to multi-line comments, you’ll need a block format, disallowing shorthand methods. Consider this:
/ This is a Multi-line comment/
/
* This is a
* Multi-line
* comment
*/
Clear and thoughtful code comments provide explicit explanations of different code blocks, akin to Zend framework’s “.zend” documentation files. This non-shorthand approach aids teammates’ understanding and serves as self-explanatory documentation. Comments also act as helpful notes for future reference, ensuring clarity when the code speaks in a detailed manner.
Documentation Blocks
Documentation blocks occupy a significant role in file structure. They contain details about the class in a file and its members, in essence acting as vital helper methods for understanding the code. Here, you use a similar syntax to JavaDoc, enabling auto-generation of documentation files.
A block typically patterns an entity like this:
/*
* Class to retrieve products
* @package myPackage
* @subpackage products
*/
class ProductClass { // Class properties and methods go here }
Including doc blocks helps to maintain top-tier code quality and readability. Particularly in templates where PHP code is embedded within HTML, doc blocks can be a lifeline in understanding the code. Document wisely, showing respect towards your code and your peers! This is crucial as it aids in an enterprise-wide understanding of the code, enhancing collaboration.
PSR-1: Basic Coding Standard
Class names and Namespace Declarations
Declaring namespaces and class names step-up your PHP coding style. The consistent namespace declaration rules, tied closely with the concept of vendor namespace–such as Vendor\Project_Name, mandate that:
- The declaration is the first statement in a file, succeeded by a blank line for method declaration.
- The namespace, following zend style, name starts with a capital letter and follows camel case convention.
Refer to this example:
namespace MyCompany;
use FooClass;
use BarClass as Bar;
class MyClass
{
// Class properties and helper methods go here
}
namespace MyCompany;
use FooClass;
use BarClass as Bar;
class MyClass
{
// Class properties and helper methods go here
}
The primary purpose of namespaces is categorization, promoting efficient file organization–a form of grouping. Class names, always adhering to UpperCamelCase, are typically descriptive nouns while also incorporating interface patterns.
Remember these basic yet crucial conventions, as they pave the way for clearer, more manageable PHP coding. Every name has meaning, and in PHP, a name signs the responsibility.
Constant Names & Function Names
In PHP, constants like STUFF_LEVEL are in UPPERCASE with underscores. Use const
for better performance. Functions, like my_module_function_name(), are lowercase with module prefixes to avoid collisions. Choose meaningful names for clarity, favoring SetValue() over SV(). Follow uppercase for acronyms and prioritize readability, enhancing code interpretation.
PSR-2: Coded with Style
File Formatting (Line Length and Indentation)
File formatting, specifically line length and indentation, matters in PHP. Plan your lines such that they do not exceed 80 characters. In line with PSR-12 specifications, this ensures alignment and readability, facilitating easy location of specific code blocks for developers. You may also break up your code into several lines for optimal readability when necessary.
// Good
$welcome = 'Hello, World!';
// Good, but should be avoided if possible
$long_variable_name = 'This is a very long line that may exceed the 80 character limit';
// Bad
$really_long_variable_name = ‘This is an even longer line that significantly goes past the 80 character limit and ideally, should be split into multiple lines’;
Indentation, as the rule of thumb goes, ought to be handled using tabs at the beginning of lines, and whitespace mid-line for alignment. Doing so provides clarity, demarcates logical blocks of code, and leads to a more profound understanding of a function or method. Removing newline at the end of files also eliminates the risk of “header already sent” errors and XHTML/XML validation issues, and further specifies metadata about indentation and alignment.
function myFunction()
{
//Variables declaration
$hello = 'Hello';
$world = 'World';
// Echo the variables
echo $hello;
echo $world;
}
By mastering file formatting aligned with the right specifications, you set the stage for code that’s clean, elegant, and easy to navigate – it’s the path to code Nirvana!
Spaces between Tokens and Placement of Braces
In PHP style, maintain one space around tokens, with exceptions for parentheses. Ensure clarity with spaces around equals and less than signs. Avoid spaces near parentheses, except between function name and opening parenthesis.
The placement of curly braces follows two main rules around control structures and the closing bracket:
- Braces should never be positioned on the same line as the control keyword, except for control structures in the style of a single-line conditional.
- Always align opening and closing braces, where the closing parenthesis and opening brace should be placed together.
For example, given a if condition,
if ($condition) { // Your code here } else { // Your code here }
An incorrect way to write it would be with extra spaces and misplaced closing tag:
if ( $condition ) {
// Your code here }
else
{ // Your code here
}
Instead, it should look like:
if ($condition)
{
// Your code here
}
else
{
// Your code here
}
Remember, using a backslash ( \ ) for special characters and wrapping variables within curly braces are recommended. Mastering the art of spacing, placing braces correctly, control keywords, avoiding unwanted white spaces with the closing tag, and parenthesis placement paves the way for code that’s almost akin to poetry.
Dealing with PHP Operators
Comparison Operators in PHP
PHP offers a buffet of comparison operators for you to choose from. Among them are == and === operators, which perform loose and strict comparisons respectively. The intent behind using these specific operators determines which is most suitable for each operation. The ‘==’ compares only value, not type, allowing for possible incorrect identifications if the variable type is not considered, for example:
```
if($_POST['user_id'] == '1234') { echo 'Hello Special user'; }
```
On the other hand, ‘===’ compares both value and type, enhancing the program’s logic and decreasing the probability of logic errors. An example of this stricter equivalence can be seen here:
```
if(IsThisAdmin($_POST['user_id']) === true) { echo 'Hello Admin'; }
```
When dealing with PHP comparison operators, it’s all about understanding the difference and the context. Always remember – when in doubt, always opt for strict comparisons! This is key to avoid potential errors in your PHP code.
Logical and String Operators
Logical operators in PHP bind multiple conditions together. The || “or” operator is discouraged due to its similarity to number ’11’ and its occasional incorrect usage, which is why it’s recommended to use the control keywords && or AND. Additionally, always preface and follow ‘!’ with a space for clarity to clearly indicate the intent of this operation.
if ($coeffe || $sugar) // Not recommended
if ($coffee && $sugar) // Recommended
if (!$morning) // Correct
Complex expressions might sometimes be more readable with control keywords than with their counterparts.
String operators are responsible for concatenating two strings. Just add a ‘.’ character for this.
$msg = 'Good' . ' morning!'; // Correct
$msg = 'Good' . ' morning!'; // Correct
Remember that control statements should have one space between the control keyword and opening parenthesis to distinguish them from function calls.
Mastering Logical and String Operators is like learning to play chords on a guitar. A little practice, and you’d be struck by how harmonious your PHP code sounds! Additionally, while structuring your code, be thoughtful and avoid making incorrect assumptions.
Control Structures in PHP
If, Elseif, Else, and Switch Case
Mastering control structures is a quintessential part of PHP coding. Amongst these, if, elseif, else, and switch cases hold significant intent. Following is the correct way to use if, elseif, and else: if ($condition1 || $condition2){action1;} else if ($condition3 && $condition4){action2;} else {defaultaction;}. Note a space must exist between the control keyword and opening parenthesis, to distinguish them from function calls.
The correction above from ‘elseif’ to ‘else if’ improves readability and is consistent, hence decreasing the likelihood of introducing logic errors.
Switch statements provide a neater mechanism for complex condition handling compared to multiple if clauses. Here’s an example:
switch($condition) { case 1: action1; break; case 2: action2; break; default: defaultaction; }
Ensure there’s a comment such as // no break intentional when fall-through is intentional in a non-empty case body—this will avoid any incorrect intention in the code.
Control structures are the wheel that steer your code in the desired direction. Learning to leverage these control structures effectively will help ensure smoother coding journeys. Strap on your seatbelt, and let’s go on a coding ride!
For, Foreach and While loops
Loops are efficient tools that spare you repetitive tasks in PHP coding. The control keywords for, foreach, and while are employed most commonly in loops.
A for loop adheres to this format, and do note the recommended space between the control keyword and opening parenthesis:
for ($i = 0; $i < 10; $i++) { / for body / }
for ($i = 0; $i < 10; $i++) { / for body / }
To work with arrays and minimize logic errors, choosing a foreach loop often produces cleaner code:
foreach ($array as $key => $value) { / foreach body/ }
foreach ($array as $key => $value) { / foreach body/ }
Last but not least, meet the while loop:
while($condition) { / Code to be executed / }
while($condition) { / Code to be executed / }
Remember; loops can be nested, but if you sink to $z, revising your code is advised for better readability, part of avoiding logic errors.
Embrace loops for they’re your friends in a universe of repeated actions. True enough, a cup of tea for loops is indeed repetitive tasks!
Implementing PHP Functions and Classes
Function Definition and Declaration
Enhance PHP code readability with lowercase, underscored function names like get_message(). Emphasize clear parameter order, type hints, and defaults (e.g., func($arg1, $arg2 = ‘asc’, $arg3 = 100)). Utilize spread operators for efficient argument handling. Maintain a precise syntax for function declarations, ensuring a smooth coding voyage.
Class Properties and Methods
Class properties and methods form the backbone of object-oriented programming in PHP. One essential part of writing methods is the method declaration, where you specify its name, parameters and visibility. According to PHP coding standards, visibility MUST always be included. To avoid ambiguity, refrain from using the underscore prefix for private or protected properties. For an effective instance, let me share the appropriate example below:
namespace MyCompany\Model;
class User{
public $var1;
protected $var2;
private $var3;
}// EOF
When declaring functions, use the spread operator for packing arguments, and avoid spaces between the closing parenthesis and the colon in return types. Ensure method names are clear, follow visibility practices, type hinting, and naming conventions. When using multiple modifiers, maintain a specific order. Class properties and methods serve as the architectural blueprint for object-oriented projects; adhere to standards for code clarity and evolution. Use ‘else if’ for readability and consistency, maintaining one space between control keywords and opening parenthesis. Build your code wisely!
Modern PHP Tools
The Use of PhpStorm for PHP Coding
PhpStorm, a popular IDE for PHP, enhances coding with features like code completion and debugging. It simplifies testing and deploying, making PHP development efficient. However, mastering PHP coding standards is crucial for security and functionality. Equip yourself with knowledge and modern tools to code like a pro!
Utilizing Beautify PHP for Code Aesthetics
Beautify PHP, as the name suggests, is all about enhancing your PHP code’s aesthetics. Its main job is auto-formatting your code to improve readability and maintainability.
Whether it’s converting spaces to tabs, beautifying and minifying codes, or automatically inserting PHPDoc Blocks, Beautify PHP has got you covered.
Remember, coding is not just about efficiency; aesthetics matter too. And with Beautify PHP, you’ve got an interior decorator for your code. Let’s make beautiful code.
Encoding with PHP Best Practices
Dealing with Global Variables
Global variables, though tempting, are to be used sparingly. Their incorrect usage, usually due to logic errors, can lead to unexpected side effects – making debugging a nightmare. Reflecting on the idea of improperly declaring multi-part names like `$my_movies = array( ‘Slumdog Millionaire’, ‘Silver Linings Playbook’, ‘The Lives of Others’, ‘The Shawshank Redemption’)` can serve as cautionary tales. Here’s how to correctly declare them:
- global $global_var1;
- global $global_var1;
Use this syntax to circumvent the errors of declaring multiple global variables on one line. For consistency, variable names should start with a single underscore followed by the theme name and another underscore. Avoid mistakes like `$welcome_Message`, `$Welcome_Message` and `$WELCOME_MESSAGE` not all being lowercase. Remember that incorrect capitalization in variables, much like in `welcome_Message`, `Welcome_Message` and `welcome_message`, can lead to logic errors.
While global variables seem convenient at first, they often pose potential pitfalls. Use them judiciously —always be vigilant of potential logic errors when juggling with global variables. They might turn out to be jokers!
Regular Expressions and Error Reporting
PHP is proficient at handling regular expressions and error reporting, pillars in robust coding.
Regular expressions (regex) are potent tools used to find or match patterns in strings. Use them judiciously as they can become convoluted.
preg_match('/abc/', 'abcdef'); // return 1
preg_match('/123/', 'abcdef'); // return 0
Error reporting in PHP is paramount for the development of a high-quality application. Aim to develop code that is error-free under E_ALL setting. Use ini_set('display_errors', '1');
at the beginning of your script to display all errors, warnings and notices.
Remember, regular expressions are your powerful searchlights and error reporting your safety harness. Embrace them and watch your coding caliber skyrocket. Coding safe and smart is the modern coder’s mantra.
Top PHP-FIG Approved Frameworks
Symfony: A Comprehensive PHP Framework
Excelling in PHP coding becomes a breeze with the right framework. Symfony is one of the PHP-FIG approved frameworks that stands out in the crowd, showing similarities to other renowned frameworks like zend framework and CodeIgniter.
Used by industry giants like Magento and Drupal, Symfony is not only comprehensive but also highly flexible. Its innovative bundles system, inspired in part by best practices from CodeIgniter, Drupal, Horde, Pear, PSR-1, PSR-2, Symfony, and WordPress, allows for effortless code reuse across projects. Moreover, it fosters stringent testing environments by offering functional and unit testing mechanisms.
Respecting the PEAR coding standards, Symfony embraces the DRY (Don’t Repeat Yourself) principle, pushing developers to maintain clean, performant, and reusable code – a practice that further mirrors the lauded CodeIgniter and zend traditions. With symfony on your side, you’ve got a powerful accomplice to thrive in the modern PHP landscape. Step up and harness the Symfony framework, and as CodeIgniter endorses, remember, a good framework is half the work done!
Laravel: An Intuitive Framework for Modern Web Application
Laravel, an acclaimed PHP-FIG framework, draws inspiration from CodeIgniter and PEAR, ensuring an elegant syntax and developer-friendly experience. With MVC support and an efficient artisan CLI, it aligns with PEAR standards, promoting performance and security. Laravel’s robust community and diverse packages make it a powerful tool for crafting modern, scalable web applications. Embrace Laravel for an enchanting journey in the PHP coding realm!
FAQ – Frequently Asked Questions
What are PHP Coding Standards?
PHP Coding Standards, influenced by frameworks like CodeIgniter and PEAR, provide guidelines for clean, readable code. Following these standards enhances collaboration, reduces errors, and improves code readability. Embrace them, such as PEAR Coding Standards, as your guide for crafting masterful, error-resistant code.
Why is PHP style guide important?
A reliable PHP coding style guide, inspired by leaders like CodeIgniter and Pear, ensures consistency in your projects. It emphasizes clear variable names, discourages common pitfalls, and promotes elegant code. Following such a guide enhances debugging, collaboration, and code reviews, fostering efficient and maintainable PHP code, akin to the best practices of CodeIgniter and Pear standards. Embrace it as your passport to better coding!
How can I improve my PHP coding skills?
Master PHP basics like syntax, operators, and arrays, then delve into advanced topics like OOP and regular expressions. Explore frameworks such as Laravel and CodeIgniter, follow coding standards like Pear, and practice on platforms like HackerRank. Engage in PHP communities, embrace code reviews, and embrace the learning journey. Happy coding!