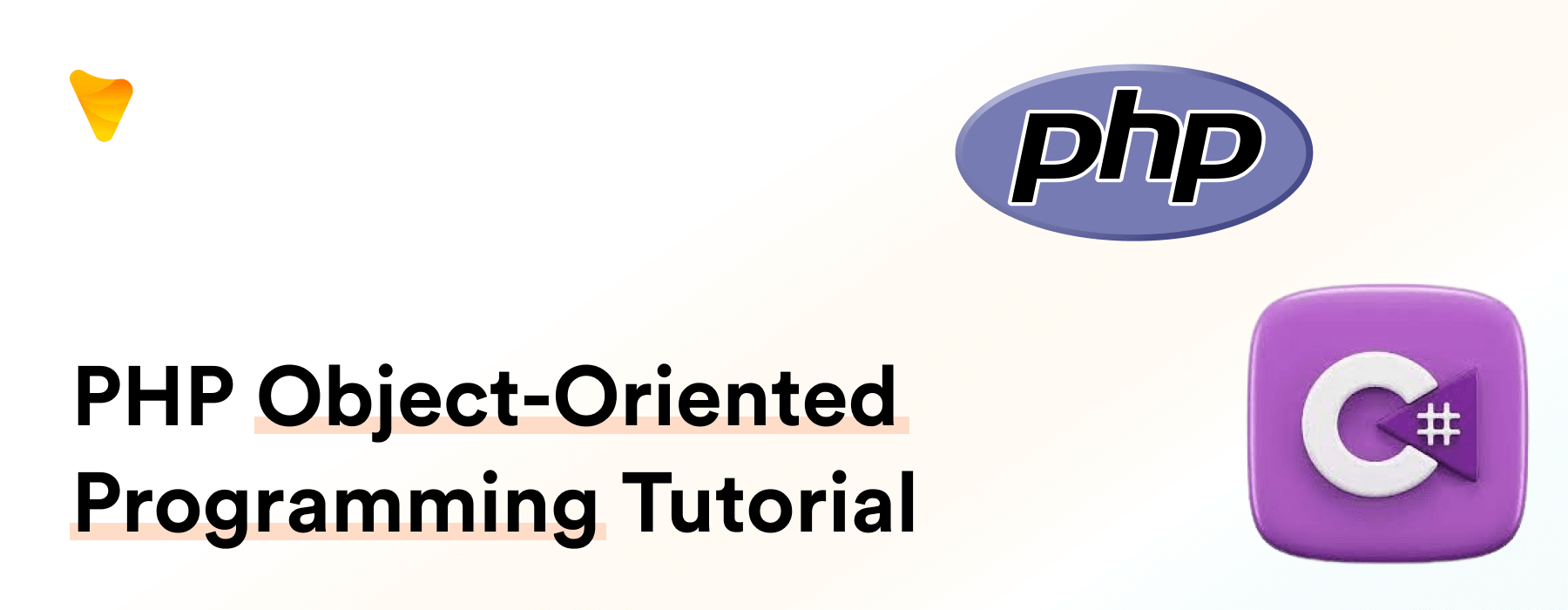
The Complete Object-Oriented Programming Tutorial for PHP
Introduction to Object-Oriented Programming in PHP
What is Object-Oriented Programming?
Object-Oriented Programming (OOP) focuses on objects and data rather than actions and logic, prioritizing efficient object manipulation in languages like PHP. Understanding OOP concepts is crucial in PHP, a language robustly supporting these features. Embracing OOP brings efficiency, manageability, and structure to coding, paving the way for scalable, maintainable, and reusable applications. New programming languages are evolving, inheriting and extending the benefits of OOP.
Why Use Object-Oriented Programming in PHP?

OOP in PHP stands out for multiple reasons. PHP’s OOP enhances code readability, reusability, and simplifies managing large applications, making it a preferred choice for programmers. It’s scalable, allowing for easy growth in complex web applications without significantly affecting the entire codebase. Moreover, it simplifies testing and debugging, thereby reducing the likelihood of errors. As highlighted by Adebayo Adams, OOP continues to stay relevant and millions of programmers use it for web application development. It’s a must-know for every aspiring PHP developer.
The Core Pillars of OOP in PHP
Objects and Classes: The Foundation Blocks
In the world of OOP, objects and classes are the building blocks. A class, also called a ‘childclass,’ acts like a blueprint for objects, defining their attributes and interactions. Inside, the ‘class constructor’ (or ‘__construct’ method) initializes objects, using the flexible ‘data type var’ in PHP for improved type safety. Objects represent real-world entities with values, so understanding the difference between objects and child classes is crucial in OOP.
Understanding Inheritance in PHP
In PHP, inheritance simplifies creating new classes by inheriting properties and methods from existing ones. Use the “implements” keyword for this. Like humans inherit traits, classes gain attributes, promoting code reuse and readability. Create a ‘Mammal’ parent class with common methods for efficient code sharing. Incorporate the parent class constructor for depth. For instance, a ‘Furniture’ parent class with a unique constructor can be used in a ‘Sofa’ child class to avoid redundancy and optimize code, showcasing PHP’s flexibility across operating systems and in command-line scripting.
Encapsulation: Shielding Your Data
Encapsulation forms PHP’s mechanism of bundling data with related functionality. PHP abstraction unites data and functions, concealing internal details. Access only essential details for task execution, enhancing security. This approach takes encapsulation one step further by incorporating “private” or “protected” access modifiers. Consider it a security measure that binds together critical parts of your code to prevent unauthorized access, boosting application safety and maintainability. Introducing the concept of Late Static Binding here, abstraction allows for internal changes—like driving a car without needing inside mechanism knowledge—without impacting other parts of your code.
Demystifying Polymorphism in PHP
Polymorphism in PHP, originating from Greek words ‘poly’ (many) and ‘morph’ (forms), adds versatility to your code. Polymorphism in object-oriented programming, akin to big data, enables seamless interchangeability of diverse class objects. It brings flexibility and maintainability to code, eliminating the need for constant data redeclaration. Using a shared parent class, like Apache Hadoop or Spark in big data, streamlines processes, enhancing efficiency and organization in PHP.
Getting to Grips with PHP Classes and Objects
Defining PHP Classes
In PHP, creating classes involves using the “class” keyword, choosing a name, and defining variables with “var” for data members. Understanding this is crucial for Object-Oriented Programming (OOP), akin to mastering the “final” keyword. Structuring PHP files effectively is vital, as discussed in many articles. For instance, creating a “Car” class with variables like “color” and “manufacturer” ensures maintainability. Adding methods such as “accelerate” and “brake” makes code reusable. Stay updated on PHP by subscribing to the latest articles for self-taught developers like Adams.
Creating and Using Objects in PHP
Creating and using objects in PHP is straightforward once you grasp the constructor concept and the broader scope of object-oriented programming (OOP). Begin by defining a class, like “Furniture” or “Person,” and use a constructor with the “new” keyword to generate objects. Objects, such as “Person” or “Furniture,” represent unique instances of their respective classes. Incorporating constructors and destructors efficiently manages these objects. Objects can have distinct property values while sharing common methods, allowing your code to mimic real-world behaviors. Practical examples, like creating a new “Person” object and demonstrating object-oriented programming with a while loop, illustrate the efficiency and practicality of OOP in PHP. This is a crucial step in understanding classes and the potential output of objects, contributing to efficient and readable code.
Advanced Concepts of OOP in PHP
Magic Methods and Their Uses
PHP magic methods, like __toString() for object-to-string conversion and __call() for dynamic method invocation, add versatility. Examples include __serialize() and __wakeup() for object serialization. These methods, like a Furniture class’s __construct($price, $type), enhance PHP’s object-oriented programming by enabling customization and flexibility, making code more robust and user-friendly.
Digging into Static Properties and Methods
Static properties and methods in PHP, declared with the ‘static’ keyword, enable direct access without creating an instance of the class. Use the class name and double colon (::) to call them. They provide shared functionality across all instances, allowing changes to propagate dynamically. Unlike ‘define()’, ‘const’ is case-sensitive by default, preserving constancy like a static property.
Embracing Abstract Classes and Methods
Abstract classes and methods act as blueprints, providing a structured template for child classes. In PHP, the “interface” keyword helps achieve abstraction, allowing essential details for specific tasks while hiding internal complexities. Abstract classes, like ‘Shape,’ enforce uniformity, offering a flexible and maintainable code foundation for advanced abstraction, ensuring reusable structures.
The Practical Side of PHP OOP
Object-Oriented Programming Principles in PHP
PHP OOP: Encapsulation (class bundling), Inheritance (parent-child classes), Polymorphism (interchangeable objects). Learn core principles for powerful coding. Implementing these principles makes your PHP code organized, systematic, and flexible, and promotes code reusability. Besides, they introduce a more simple and clear approach to software development, shaping the future of PHP programming.
Comparing Object-Oriented and Procedural Code
Procedural code follows a step-by-step approach with separate functions for data and actions. In contrast, OOP combines data and functions into objects for a more organized and reusable structure. OOP’s DRY principle reduces code modification when adding features, making it advantageous for complex projects. Choose based on your project’s needs.
Exception Handling in OOP PHP
Exception handling in PHP, crucial for server-side development, lets you manage errors gracefully. Using “try,” “catch,” and “finally” blocks, you can execute code under watchful eyes without disrupting the flow. Caught exceptions in the “catch” block enable clean error messages. PHP supports custom exception classes for precise error control. Server setup requires PHP, a web server, and a browser.
Harnessing the Power of OOP in PHP: A Case Study
Implementing Inheritance in PHP: A Walkthrough
Unleash PHP inheritance for efficient, reusable code! Explore creating a ‘Dog’ class that inherits from a ‘Animal’ class, utilizing ‘extends’ for code reusability. This relationship allows access to parent class methods and properties. Discover how to override methods for specialized behavior, enhancing cleaner, DRY-compliant coding. Dive into ‘makeSound’ variations for ‘Dog’ and ‘Cat,’ and elevate your PHP skills with powerful, streamlined code.
Encapsulation in PHP: A Practical Example
Encapsulation in PHP, demonstrated practically, begins with the establishment of a class. Consider a ‘BankAccount’ class with properties for ‘balance’ and methods for ‘deposit’ and ‘withdraw’. You may want to keep ‘balance’ private – inaccessible from outside the class. Here’s how you can do it:
class BankAccount {
private $balance;
public function deposit($amount) {
$this->balance += $amount;
}
public function withdraw($amount) {
if ($this->balance >= $amount) {
$this->balance -= $amount;
} else {
return "Insufficient funds.";
}
}
}
In the example above, the $balance
property is marked as private
, so it isn’t accessible directly from outside the class. Instead, we establish public methods deposit()
and withdraw()
to modify $balance
, keeping it safe from unwanted external manipulations. This approach ensures improved security and code robustness.
Wrapping up: Closing Thoughts on OOP in PHP
Advantages of OOP: Better Organization and Easier Maintenance
Explore PHP Inheritance for cleaner, DRY code. Benefit from OOP advantages like organized code, scalability, and code reusability. Simplify debugging and enhance performance for robust software development in PHP.
Future of OOP in PHP: What Lies Ahead?
PHP’s OOP future looks promising, with evolving support and frameworks like Laravel embracing OOP principles. The arrow operator enhances object manipulation, making code more flexible and maintainable. As PHP development advances, a strong grasp of OOP, including the arrow syntax, is essential for growth. OOP’s prominence in PHP seems enduring, shaping the landscape for years to come.
Frequently Asked Questions (FAQs)
How Do I Create a Class in PHP?
Creating a class in PHP is straightforward. Begin with the keyword ‘class’, followed by a name of your choice, and then a set of curly braces {}. Inside these, you define properties and methods for your class. Here’s an example:
class Car {
// Class properties
var $color;
var $manufacturer;
// Class methods
function setColor($color) {
$this->color = $color;
}
function setManufacturer($manufacturer) {
$this->manufacturer = $manufacturer;
}
}
In this example, ‘Car’ is a class with properties ‘color’ and ‘manufacturer’, and methods to set these properties. This class can now be used to create multiple ‘Car’ objects.
What Is the difference between Public, Private, and Protected Members?
Public, private, and protected members are access modifiers in PHP, regulating the accessibility of class properties and methods.
-public members are accessible from anywhere – inside the class, outside, and from child classes. This is the default visibility in PHP.
-protected members can only be accessed within the class itself and by child classes (classes that extend it). They are not accessible from outside the class.
-private members can only be accessed within the class that defines them. Even child classes cannot access private members.
In essence, these modifiers provide control over where and how our class properties and methods are accessed and modified, thereby enhancing code security and robustness.
What Are Magic Methods in PHP?
Magic methods in PHP are special methods that provide hooks to execute code automatically at specific moments. These predefined methods always start with a double underscore “__”. Here are some commonly used ones:
- __construct(): Executes when an object is created.
- __destruct(): Executes when an object is destroyed.
- __get(): Invoked when you try to access a non-existent or inaccessible property.
- __set(): Called when you try to set a value for an inaccessible or non-existent property.
- __call(): Triggered when an inaccessible or non-existent method is invoked.
- __toString(): Enables a class object to be converted to a string.
These magic methods pave the way for more expressive and powerful object-oriented programming in PHP.
What is Inheritance in OOP PHP?
Inheritance in OOP PHP refers to the mechanism where you can create new classes based on an existing class. The new class inherits all the public and protected properties and methods of the existing class, promoting code reusability and reducing redundancy.
Here is a syntax example:
class Automobile { ... }
class Car extends Automobile { ... }
The ‘Automobile’ class is the base or parent class and ‘Car’ is the derived or child class. ‘Car’ inherits the accessible members of ‘Automobile’. Inheritance forms a crucial aspect of OOP in PHP, contributing significantly to its flexibility and maintainability.